Well, I’ve been fuming for the past few weeks over something, and I’ve just now gotten around to doing something about it.
I went back to my dorm room the other day, and a few buddies followed me in. One of them was carrying a camera; he was filming me for whatever reason (he’s weird like that). Anyway, I sat down at my computer and unlocked it (brought it out of the screensaver with my password) so I could begin studying for the upcoming finals (the next morning). Soon as I did, the dude with the camera behind me smiled and said, “Now I’ve got your password.” I didn’t know what to do. My computer password is the most secure password I have: alpha, numeric, symbol, and 10 characters long. I use that VERY secure password for the things that I REALLY need secured: computer data, and banks. The first former would be bad, but the later would be worse. If he knows that password, he can literally bankrupt me. Oh shit.
So, something had to be done; I needed to evolve the way I secure things. I’m in college now, so I should probably do it anyway. I need grown-up security.
I can’t really thank the camera kid for pushing me to change my password protocol, but it certainly has helped me develop a better way of securing my confidential information.
First, I needed to obliterate that password from existence. I needed a new password–no, I needed several new passwords. I created eight passwords for varying levels of security. I’ve got passwords setup for banks, passwords for forums, and passwords for other stuff in between.
Second: Wait. I need more than eight passwords. If someone discovers one of my passwords again, they’d have access to everything! No, I need unique passwords for everything. So, I hybridized the whole process.
I decided to create 8 generic passwords (alpha, numeric, symbolic) at a length of 10 characters. Then I append an additional. five unique characters onto the end of those 10 characters for actual use. To create a truly random set of passwords, I wrote my first C++ application to decide on my passwords for me.
pass.c #include <stdio.h> #include <stdlib.h> #include <time.h> int main () { int rNum,i; srand ( time(NULL) ); for(i=0;i<6;i++) { rNum=rand() % 62 + 1; if (rNum==1) puts ("a"); else if (rNum==2) puts ("b"); else if (rNum==3) puts ("c"); else if (rNum==4) puts ("d"); else if (rNum==5) puts ("e"); else if (rNum==6) puts ("f"); else if (rNum==7) puts ("g"); else if (rNum==8) puts ("h"); else if (rNum==9) puts ("i"); else if (rNum==10) puts ("j"); else if (rNum==11) puts ("k"); else if (rNum==12) puts ("l"); else if (rNum==13) puts ("m"); else if (rNum==14) puts ("n"); else if (rNum==15) puts ("o"); else if (rNum==16) puts ("p"); else if (rNum==17) puts ("q"); else if (rNum==18) puts ("r"); else if (rNum==19) puts ("s"); else if (rNum==20) puts ("t"); else if (rNum==21) puts ("u"); else if (rNum==22) puts ("v"); else if (rNum==23) puts ("w"); else if (rNum==24) puts ("x"); else if (rNum==25) puts ("y"); else if (rNum==26) puts ("z"); else if (rNum==27) puts ("A"); else if (rNum==28) puts ("B"); else if (rNum==29) puts ("C"); else if (rNum==30) puts ("D"); else if (rNum==31) puts ("E"); else if (rNum==32) puts ("F"); else if (rNum==33) puts ("G"); else if (rNum==34) puts ("H"); else if (rNum==35) puts ("I"); else if (rNum==36) puts ("J"); else if (rNum==37) puts ("K"); else if (rNum==38) puts ("L"); else if (rNum==39) puts ("M"); else if (rNum==40) puts ("N"); else if (rNum==41) puts ("O"); else if (rNum==42) puts ("P"); else if (rNum==43) puts ("Q"); else if (rNum==44) puts ("R"); else if (rNum==45) puts ("S"); else if (rNum==46) puts ("T"); else if (rNum==47) puts ("U"); else if (rNum==48) puts ("V"); else if (rNum==49) puts ("W"); else if (rNum==50) puts ("X"); else if (rNum==51) puts ("Y"); else if (rNum==52) puts ("Z"); else if (rNum==53) puts ("1"); else if (rNum==54) puts ("2"); else if (rNum==55) puts ("3"); else if (rNum==56) puts ("4"); else if (rNum==57) puts ("5"); else if (rNum==58) puts ("6"); else if (rNum==59) puts ("7"); else if (rNum==60) puts ("8"); else if (rNum==61) puts ("9"); else if (rNum==62) puts ("0"); else if (rNum==63) puts ("`"); else if (rNum==64) puts ("~"); else if (rNum==65) puts ("!"); else if (rNum==66) puts ("@"); else if (rNum==67) puts ("#"); else if (rNum==68) puts ("$"); else if (rNum==69) puts ("%"); else if (rNum==70) puts ("^"); else if (rNum==71) puts ("*"); else if (rNum==72) puts ("("); else if (rNum==73) puts (")"); else if (rNum==74) puts ("-"); else if (rNum==75) puts ("="); else if (rNum==76) puts ("_"); else if (rNum==77) puts ("+"); else if (rNum==78) puts ("["); else if (rNum==79) puts ("{"); else if (rNum==80) puts ("]"); else if (rNum==81) puts ("}"); else if (rNum==82) puts (""); else if (rNum==83) puts ("|"); else if (rNum==84) puts (";"); else if (rNum==85) puts (":"); else if (rNum==86) puts ("'"); else if (rNum==87) puts ("""); else if (rNum==88) puts (","); else if (rNum==89) puts ("<"); else if (rNum==90) puts ("."); else if (rNum==91) puts (">"); else if (rNum==92) puts ("/"); else if (rNum==93) puts ("?"); } return 0; }
There’s supposed to be 94 characters on a standard keyboard, but I could only find 93. Anyway, this will give me some nice base, generic passwords. Supposedly, these 15-character, unique passwords from 93 characters will be yield 93^15 = 336,700,862,051,614,156,853,075,413,557 different possibilities. I know that rainbow tables are supposed to be impressive at cracking hashes (if they ever get access to one), but those odds leave me relatively satisfied.
Another thing I began to think about is file encryption. I don’t want to encrypt my entire hard disk because if anything in grub or the kernel gets royally fucked, I can’t just plug in the disk as a secondary to another working computer and copy my files over. Instead, I was looking for a way encrypt a file so that it could only be viewed if the right password were provided. After a little bit of searching, I found my answer in GnuPG (GNU Privacy Guard). You can spend a few hours trying to comprehend the concepts and mathematics behind the encryption, but all I know is that what I wanted was symmetric cipher encryption.
Let’s say that you have a file “foo.txt” with the contents of “bar”. If you run the command:
gpg -c foo.txt
It will prompt you for a passphrase. Type it twice (for verification purposes), and gpg will create a file “foo.txt.gpg”. This file contains the text:
^�
^D^C^C^B^S^Uv^G��F7`�!^E</�^K^A �^�H^��^�B�-�y^��^.’��^��!/^[%
…not very reveling of “bar,” is it? But, if I run the command:
gpg -o foo.txt.decrypted -d foo.txt.gpg
…and enter the correct passphrase, I get the text “bar” back. Excellent.
Now, even if someone manages to steal my password again, they will only have access to one system. Furthermore, they will only have access to files that I deemed “not important enough to encrypt.”
Related Posts
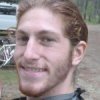
Hi, I’m Michael Altfield. I write articles about opsec, privacy, and devops ➡
Leave a Reply